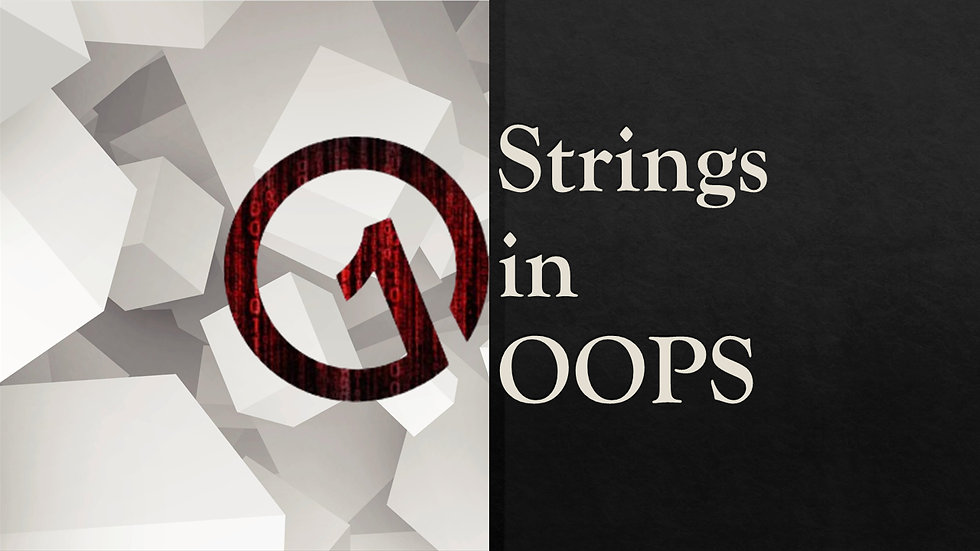
In Java, a string is a sequence of characters that can be represented as an object of the java.lang.String class. Strings in Java are immutable, which means that once a string object is created, its value cannot be changed.
String Operation -
Here are some basic examples of working with strings in Java:
Creating a string:
String myString = "Hello, world!";
Concatenating strings:
String firstName = "John";
String lastName = "Doe";
String fullName = firstName + " " + lastName;
Comparing strings:
String str1 = "hello";
String str2 = "HELLO";
boolean isEqual = str1.equalsIgnoreCase(str2);
// returns true
Finding the length of a string:
String myString = "Hello, world!";
int length = myString.length();
// returns 13
Converting a string to lowercase or uppercase:
String myString = "Hello, world!";
String lowercaseString = myString.toLowerCase();
String uppercaseString = myString.toUpperCase();
Extracting a substring:
String myString = "Hello, world!";
String substring = myString.substring(0, 5);
// returns "Hello"
Splitting a string:
String myString = "apple,banana,orange";
String[] fruits = myString.split(",");
Searching: The indexOf() method searches a string for a specified substring, while the lastIndexOf() method searches for the last occurrence of a substring.
String s1 = "Hello World";
int index = s1.indexOf("o");
// returns 4
These are just a few examples of what you can do with strings in Java. The java.lang.String class provides many more methods for working with strings, including searching, replacing, trimming, and more. Strings are an essential part of Java programming and are used extensively in many applications.
📕 Escape Character
In Java, an escape character is a character that is used to represent a special character in a string literal. The escape character in Java is the backslash (\).
Here are some of the most commonly used escape sequences in Java:
\n - newline This sequence is used to insert a newline character in a string.
Example:
String s = "Hello\nWorld";
System.out.println(s);
Output:
Hello
World
\t - tab This sequence is used to insert a tab character in a string.
Example:
String s = "Name:\tJohn";
System.out.println(s);
Output:
Name: John
\" - double quote This sequence is used to insert a double quote character in a string.
Example:
String s = "She said, \"Hello!\"";
System.out.println(s);
Output:
She said, "Hello!"
\\ - backslash This sequence is used to insert a backslash character in a string.
Example:
String s = "C:\\Program Files\\Java";
System.out.println(s);
Output:
C:\Program Files\Java
These are just a few examples of how escape characters are used in Java strings. By using escape characters, you can include special characters and symbols in your string literals that might otherwise be difficult to include.
String using literals vs new keyword
In Java, there are two ways to create a string: using string literals and using the new keyword.
String literals: A string literal is a sequence of characters enclosed in double quotes. When a string literal is assigned to a string variable, Java creates a new string object in the string pool if it does not already exist.
Example:
String s1 = "Hello World";
// creates a new string object in the string pool
String s2 = "Hello World";
// does not create a new string object, uses the same object in the string pool
new keyword: The new keyword is used to create a new string object. When a new string object is created using the new keyword, Java allocates memory for the string object on the heap, and the string object is not added to the string pool.
Example:
String s3 = new String("Hello World");
// creates a new string object on the heap
String s4 = new String("Hello World");
// creates a new string object on the heap
There are some differences between creating a string using string literals and using the new keyword. One of the main differences is that when a string literal is used, Java first checks the string pool to see if the string already exists, and if it does, the same string object is used. This can help to reduce memory usage and improve performance in some cases. On the other hand, when the new keyword is used, a new string object is always created on the heap, even if an identical string already exists in the string pool.
In general, it is recommended to use string literals whenever possible, as this can help to improve performance and reduce memory usage. However, there may be cases where using the new keyword is necessary, such as when you need to create a string object with a specific character encoding or when you want to ensure that a new string object is created each time.
📕 Different Methods of Java String
Here is a table summarizing some of the most commonly used methods of the Java String class:
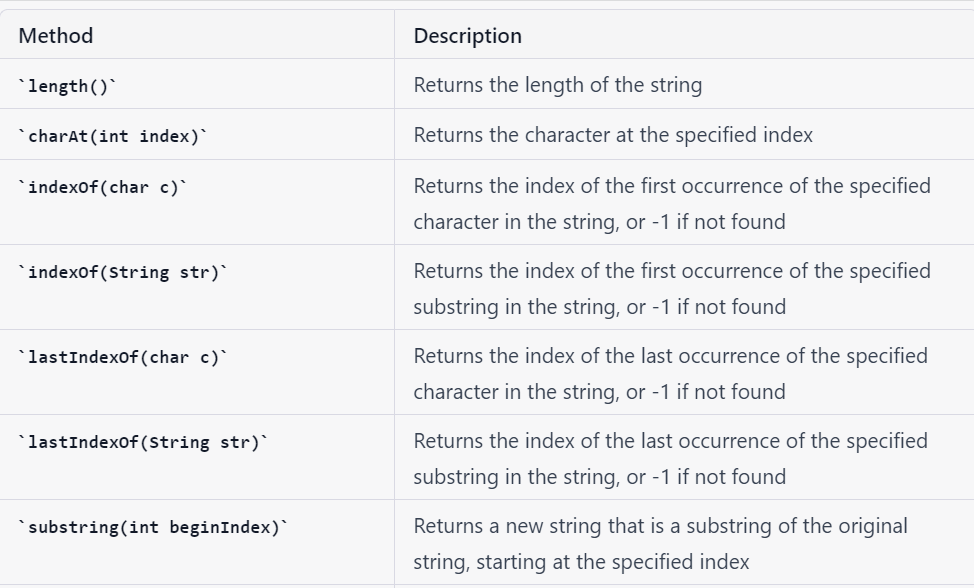

Note that this is not an exhaustive list of all the methods of the String class, but rather a summary of some of the most commonly used ones. The Java String class provides many other useful methods as well.
Thanks for reading, and happy coding!
Working with Strings in Java: Methods and Examples -> Java Access Modifiers: Understanding Public, Private, Protected and Default