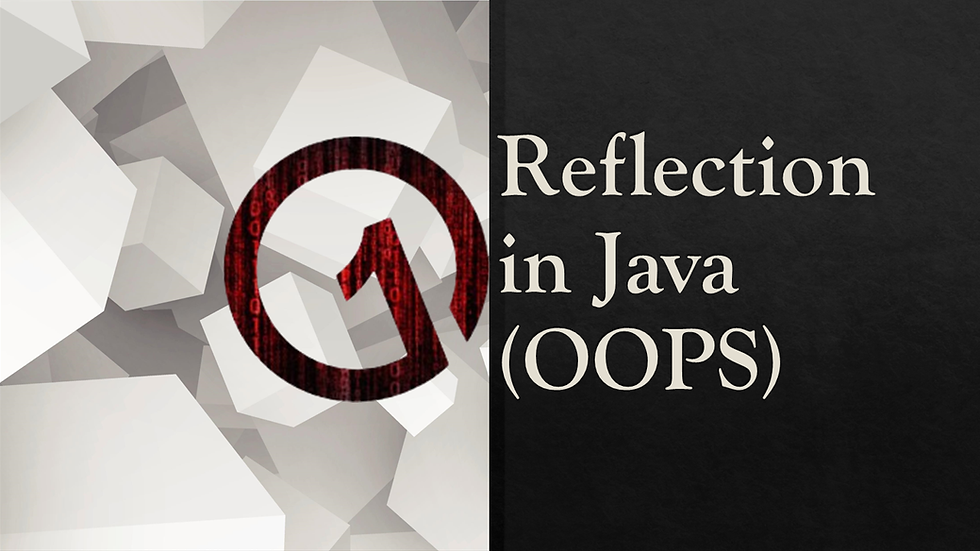
⚡ Definition
Reflection in Java is a powerful feature that allows you to inspect and modify code dynamically at runtime. It is often used to build powerful libraries and frameworks that can adapt to different environments and configurations. Here are some of the key topics you need to know about Reflection in Java, along with examples:
♦ Class Object
The Class object is the entry point for Reflection in Java. You can obtain a Class object for any class in your program using the .class operator or the getClass() method. Once you have a Class object, you can use it to inspect and modify the class definition at runtime.
Example:
Class<?> cls = String.class;
System.out.println(cls.getName());
// prints "java.lang.String"
♦ Reflection API:
The Reflection API provides a set of classes and methods for inspecting and modifying the structure of classes and objects at runtime. Some of the most important classes in the Reflection API include Class, Constructor, Field, and Method.
Example:
kotlinCopy code
Class<?> cls = String.class;
Constructor<?>[] constructors = cls.getDeclaredConstructors();
for (Constructor<?> constructor : constructors) {
System.out.println(constructor.getName());
}
♦ Accessing Fields:
Reflection allows you to access the fields of an object at runtime, even if they are declared as private. You can use the Field class to get and set the value of fields in an object.
Example:
Class<?> cls = MyClass.class;
Object obj = cls.newInstance();
Field field = cls.getDeclaredField("myField");
field.setAccessible(true);
field.set(obj, "new value");
System.out.println(field.get(obj));
♦ Invoking Methods:
Reflection also allows you to invoke methods on an object at runtime, even if they are declared as private. You can use the Method class to get and invoke methods in an object.
Example:
Class<?> cls = MyClass.class;
Object obj = cls.newInstance();
Method method = cls.getDeclaredMethod("myMethod", int.class);
method.setAccessible(true);
Object result = method.invoke(obj, 42);
System.out.println(result);
♦ Creating Objects:
Reflection also allows you to create objects of a class at runtime, even if the class does not have a public constructor. You can use the Constructor class to create new instances of a class.
Example:
Class<?> cls = MyClass.class;
Constructor<?> constructor = cls.getDeclaredConstructor(String.class);
constructor.setAccessible(true);
Object obj = constructor.newInstance("constructor argument");
System.out.println(obj);
♦ Modifying Classes at Runtime:
Reflection enables you to modify the structure and behavior of classes at runtime, including adding new fields or methods, or changing the implementation of existing methods. Here's an example of how to add a new field to a class using reflection:
Class<?> cls = MyClass.class;
Field field = new FieldBuilder()
.setName("newField")
.setType(String.class)
.setModifiers(Modifier.PUBLIC)
.build();
ClassFileTransformer transformer = new ClassFileTransformer(cls.getName(), field);
byte[] bytecode = transformer.transform();
Class<?> newClass = defineClass(cls.getName(), bytecode, 0, bytecode.length);
♦ Annotation Processing:
Reflection enables you to inspect and process annotations at runtime, which can be useful for building tools that generate code or perform analysis on existing code. Here's an example of how to obtain annotations of a class using reflection:
Class<?> cls = MyClass.class;
Annotation[] annotations = cls.getAnnotations();
These are just some of the many ways that you can use reflection in Java to inspect and modify the behavior of your code at runtime. It's a powerful feature that can be used to implement advanced functionality and to build tools that work with Java bytecode.
Thanks for reading, and happy coding!