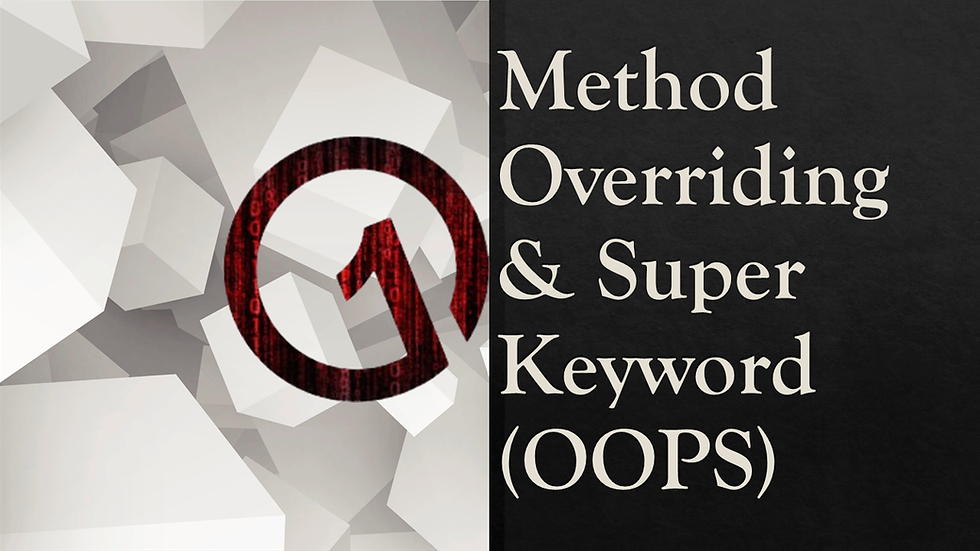
📗 Method Overriding in Java
Method overriding is a feature of Java inheritance that allows a subclass to provide its own implementation of a method that is already defined in its parent class. When a subclass overrides a method, it replaces the implementation of the method in the parent class with its own implementation.
Here are some of the key topics related to method overriding in Java, explained in detail with examples:
âš¡ Method signature and access modifier:
To override a method, the subclass method must have the same name, return type, and parameter list as the parent class method. The access modifier of the subclass method can be the same or less restrictive than the parent class method, but not more restrictive.
public class Animal {
public void makeSound() {
System.out.println("The animal makes a sound");
}
}
public class Dog extends Animal {
// Overrides the makeSound method of the Animal class
public void makeSound() {
System.out.println("The dog barks");
}
}
In this example, the Dog class overrides the makeSound() method of the Animal class with its own implementation. The method signature is the same in both classes, and the access modifier of the makeSound() method in Dog is less restrictive than the one in Animal.
âš¡ Method overriding and inheritance:
When a subclass overrides a method, the subclass method is called instead of the parent class method when the method is invoked on an object of the subclass.
Animal myAnimal = new Animal();
Dog myDog = new Dog();
Animal myOtherDog = new Dog();
myAnimal.makeSound(); // Output: The animal makes a sound
myDog.makeSound(); // Output: The dog barks
myOtherDog.makeSound(); // Output: The dog barks
In this example, we create an Animal object, a Dog object, and an Animal reference to a Dog object. When we call the makeSound() method on each object, the method in Animal is called for the Animal object, and the method in Dog is called for the Dog object and the Animal reference to a Dog object.
âš¡ Using the super keyword to call the parent class method:
When a subclass overrides a method, it can call the parent class method using the super keyword.
public class Dog extends Animal {
public void makeSound() {
super.makeSound(); // Calls the makeSound method of the Animal class
System.out.println("The dog barks");
}
}
In this example, the makeSound() method in Dog calls the makeSound() method of the parent Animal class using super.makeSound(). This allows the subclass method to include the functionality of the parent class method in addition to its own functionality.
âš¡ Method hiding:
Method hiding is a feature of Java inheritance that allows a subclass to define a static method with the same signature as a static method in its parent class. When a subclass hides a static method, the subclass method is called instead of the parent class method when the method is invoked on the subclass.
public class Animal {
public static void makeSound() {
System.out.println("The animal makes a sound");
}
}
public class Dog extends Animal {
public static void makeSound() {
System.out.println("The dog barks");
}
}
In this example, the Dog class defines a static makeSound() method with the same signature as the static makeSound() method in the Animal class. When we call the makeSound() method on the Dog class, the `makeSound' method in Dog is called instead of the makeSound() method in Animal.
âš¡ The final keyword and method overriding:
When a method in a parent class is declared final, it cannot be overridden in any subclass.
public class Animal {
public final void makeSound() {
System.out.println("The animal makes a sound");
}
}
public class Dog extends Animal {
// This will result in a compilation error: Cannot override the final method from Animal
public void makeSound() {
System.out.println("The dog barks");
}
}
In this example, the makeSound() method in the Animal class is declared final, which means it cannot be overridden in any subclass. When we try to override it in the Dog class, we get a compilation error.
âš¡ Polymorphism and method overriding:
Method overriding is a key feature of polymorphism in Java. Polymorphism allows us to treat objects of different subclasses as if they are objects of a common superclass.
public class Animal {
public void makeSound() {
System.out.println("The animal makes a sound");
}
}
public class Dog extends Animal {
public void makeSound() {
System.out.println("The dog barks");
}
}
public class Cat extends Animal {
public void makeSound() {
System.out.println("The cat meows");
}
}
Animal myAnimal = new Animal();
Dog myDog = new Dog();
Cat myCat = new Cat();
Animal[] animals = {myAnimal, myDog, myCat};
for (Animal animal : animals) {
animal.makeSound();
}
In this example, we create an array of Animal objects that contains an Animal, a Dog, and a Cat. We then loop through the array and call the makeSound() method on each object. Because of method overriding, the correct implementation of makeSound() is called for each object, allowing us to treat each object as an Animal while still calling its specific implementation of makeSound().
📗 Super Keyword in Java
The super keyword in Java is used to access members of a superclass from within a subclass. It can be used to access a superclass's methods, constructors, and instance variables. In this section, we'll go over each of these uses in detail with examples.
âš¡ Accessing Superclass Members:
The super keyword can be used to access methods or instance variables of the superclass from within a subclass. This is useful when we want to override a method in the subclass but still use the implementation in the superclass.
public class Animal {
public void makeSound() {
System.out.println("The animal makes a sound");
}
}
public class Dog extends Animal {
public void makeSound() {
super.makeSound();
System.out.println("The dog barks");
}
}
In this example, the Dog class extends the Animal class and overrides the makeSound() method. However, before printing out "The dog barks", it calls super.makeSound(), which calls the makeSound() method in the Animal class. This allows us to reuse the implementation in the superclass while still adding our own functionality.
âš¡ Using Super in Constructors:
The super keyword can also be used to call a constructor in the superclass. This is useful when we want to reuse the initialization code in the superclass's constructor.
public class Animal {
private String name;
public Animal(String name) {
this.name = name;
}
}
public class Dog extends Animal {
private int age;
public Dog(String name, int age) {
super(name);
this.age = age;
}
}
In this example, the Animal class has a constructor that takes a name parameter, and the Dog class has a constructor that takes a name and an age parameter. The Dog constructor uses super(name) to call the Animal constructor with the name parameter, which initializes the name instance variable in the Animal class.
âš¡ Using Super to Call Overloaded Constructors:
We can also use the super keyword to call an overloaded constructor in the superclass.
public class Animal {
private String name;
public Animal(String name) {
this.name = name;
}
public Animal() {
this("Unknown");
}
}
public class Dog extends Animal {
private int age;
public Dog(String name, int age) {
super(name);
this.age = age;
}
public Dog() {
super();
this.age = 0;
}
}
In this example, the Animal class has two constructors: one that takes a name parameter and one that calls the first constructor with a default value of "Unknown". The Dog class has two constructors as well: one that takes a name and an age parameter, and one that calls the Animal default constructor with super() and initializes the age instance variable to 0.
âš¡ Using Super to Access Overridden Methods:
The super keyword can also be used to access a method that has been overridden in the superclass.
public class Animal {
public void makeSound() {
System.out.println("The animal makes a sound");
}
}
public class Dog extends Animal {
public void makeSound() {
System.out.println("The dog barks");
}
public void makeAnimalSound() {
super.makeSound();
}
}
In this example, the Dog class overrides the makeSound() method, but it also has a method called makeAnimalSound() that uses super.makeSound() to call the makeSound() method in the Animal class. This is useful when we want to access the implementation in the superclass that has been overridden in the subclass.
âš¡ Using Super to Access Parent Class Members:
We can also use the super keyword to access members of the parent class that are not accessible from the subclass.
public class Animal {
private String name;
public Animal(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
public class Dog extends Animal {
private int age;
public Dog(String name, int age) {
super(name);
this.age = age;
}
public String getParentName() {
return super.getName();
}
}
In this example, the Animal class has a private instance variable name and a public method getName() that returns the value of name. The Dog class calls the Animal constructor with super(name) and initializes its own instance variable age. The Dog class also has a method called getParentName() that calls super.getName() to access the name instance variable in the Animal class.
In conclusion, the super keyword is a powerful tool in Java inheritance that allows us to reuse implementation from the superclass, call constructors, and access members that are not accessible from the subclass. By using the super keyword effectively, we can write more efficient and maintainable code.
Thanks for reading, and happy coding!
Understanding Method Overriding and the use of super Keyword in Java -> Understanding Java's Abstract Class and Abstract Methods