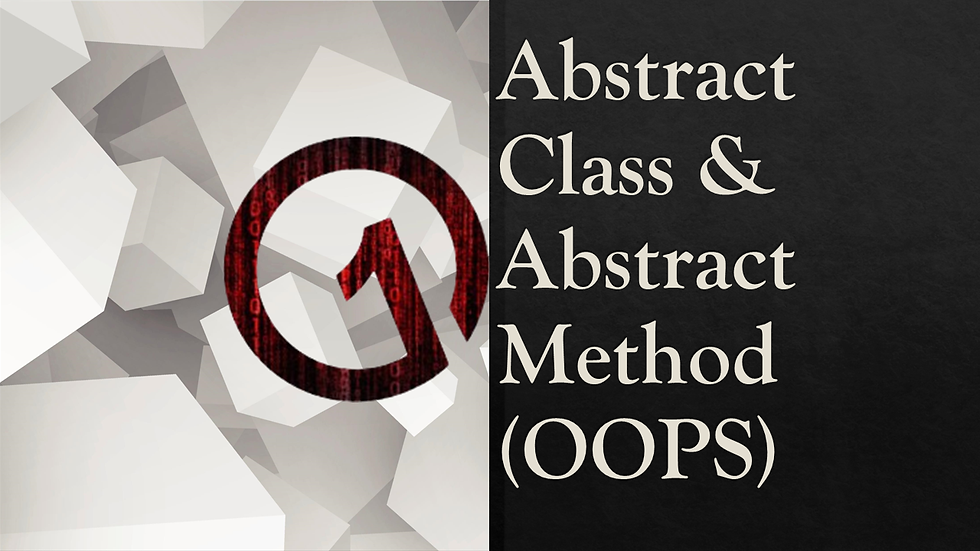
In Java, an abstract class is a class that cannot be instantiated and is designed to be subclassed by other classes. Abstract classes are used to provide a common interface for a set of related classes and to enforce the implementation of certain methods. Similarly, abstract methods are methods that are declared in an abstract class but have no implementation. Instead, they are designed to be overridden by subclasses.
Let's dive into each of these topics in more detail:
📙 Abstract Class
An abstract class is a class that is declared with the abstract keyword in its class definition. Abstract classes cannot be instantiated, but they can be subclassed by other classes. Abstract classes are used to provide a common interface for a set of related classes.
public abstract class Animal {
public abstract void makeSound();
}
In this example, the Animal class is an abstract class because it has been declared with the abstract keyword in its class definition. The Animal class also has an abstract method called makeSound() that has no implementation. This means that any class that extends the Animal class will be required to implement the makeSound() method.
✔ Abstract Method:
An abstract method is a method that is declared without an implementation in an abstract class. Abstract methods are used to enforce the implementation of certain methods in subclasses.
public abstract class Animal {
public abstract void makeSound();
public void sleep() {
System.out.println("Zzz");
}
}
public class Dog extends Animal {
public void makeSound() {
System.out.println("Woof");
}
}
In this example, the Animal class has an abstract method called makeSound() that has no implementation. The Animal class also has a concrete method called sleep() that has an implementation. The Dog class extends the Animal class and provides an implementation for the makeSound() method. Because the Dog class extends the Animal class, it is required to implement the makeSound() method.
✔ Using Abstract Classes to Provide a Common Interface:
Abstract classes can be used to provide a common interface for a set of related classes. This can be useful when you have a group of classes that have some common behavior.
public abstract class Shape {
public abstract double getArea();
public void print() {
System.out.println("This is a shape");
}
}
public class Rectangle extends Shape {
private double width;
private double height;
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
public double getArea() {
return width * height;
}
}
public class Circle extends Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
public double getArea() {
return Math.PI * radius * radius;
}
}
In this example, the Shape class is an abstract class that provides a common interface for a set of related classes. The Shape class has an abstract method called getArea() that has no implementation. The Shape class also has a concrete method called print() that has an implementation. The Rectangle and Circle classes extend the Shape class and provide implementations for the getArea() method. Because the Rectangle and Circle classes extend the Shape class, they are required to implement the getArea() method.
✔ Using Abstract Classes to Enforce Implementation:
Abstract classes can also be used to enforce the implementation of certain methods in subclasses. This can be useful when you have a group of classes that have some common behavior but also have some unique behavior.
public abstract class Animal {
public abstract void makeSound();
public void sleep() {
System.out.println("Zzz");
}
}
public class Dog extends Animal {
public void makeSound() {
System.out.println("Woof");
}
public void fetch() {
System.out.println("Fetching!");
}
}
public class Cat extends Animal {
public void makeSound() {
System.out.println("Meow");
}
public void scratch() {
System.out.println("Scratching!");
}
}
In this example, the Animal class has an abstract method called makeSound() that has no implementation. The Animal class also has a concrete method called sleep() that has an implementation. The Dog and Cat classes extend the Animal class and provide implementations for the makeSound() method. However, the Dog class has an additional method called fetch() and the Cat class has an additional method called scratch(). Because the Dog and Cat classes extend the Animal class, they are required to implement the makeSound() method, but they are also free to add additional methods that are unique to their class.
Overall, abstract classes and abstract methods are powerful features of Java that allow you to create flexible and extensible class hierarchies. By using abstract classes, you can provide a common interface for a set of related classes, enforce implementation of certain methods, and allow for unique behavior in subclasses.
Thanks for reading, and happy coding!
Understanding Java's Abstract Class and Abstract Methods -> Java Interface: Usage, Syntax and Examples.