Mutex and Producer-Consumer Problem: Synchronization Techniques in Operating Systems
- shubhangisingh453
- Mar 31, 2023
- 5 min read
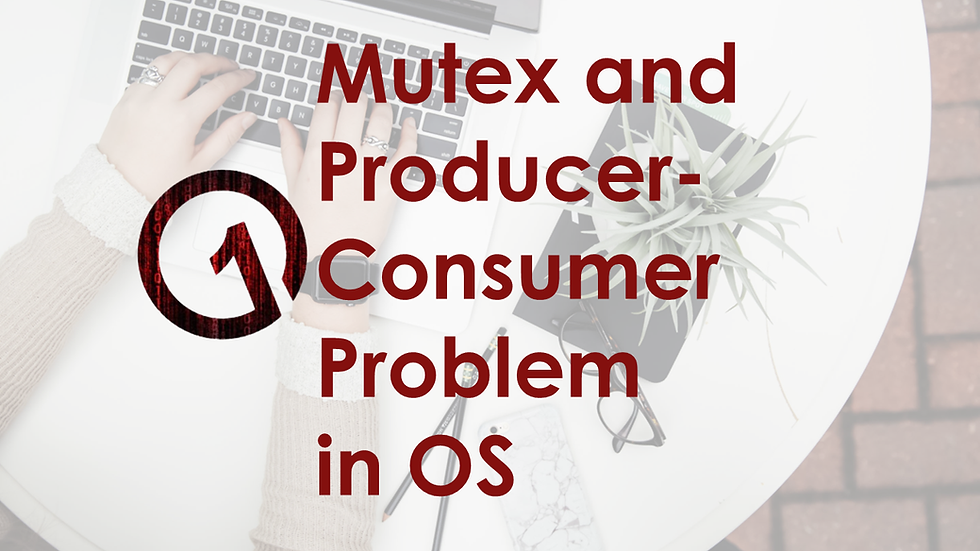
Mutex, short for mutual exclusion, is a synchronization technique used in operating systems to prevent multiple threads from accessing shared resources simultaneously. In this tutorial, we will explore what a mutex is, how it works, and its advantages in operating systems.
📗 What is a Mutex?
A mutex is a locking mechanism that provides mutual exclusion to shared resources in a multi-threaded environment. It is used to prevent race conditions that may arise when multiple threads attempt to access the same shared resource simultaneously.
📗 How Does a Mutex Work?
A mutex works by allowing only one thread to access a shared resource at a time. When a thread requests access to the resource, it must first acquire the mutex. If the mutex is not available (i.e., it is currently being held by another thread), the requesting thread will be blocked until the mutex becomes available. Once the thread has acquired the mutex, it can safely access the shared resource. When the thread is finished using the resource, it releases the mutex, allowing other threads to acquire it.
📗 Thundering Herd Problem
One issue that can arise with mutexes is the thundering herd problem. This occurs when multiple threads are waiting for a mutex to become available, and they all wake up at the same time when the mutex is released. This can lead to a performance issue, as multiple threads may contend for the mutex simultaneously, causing unnecessary context switching and reduced efficiency.
To avoid the thundering herd problem, some implementations of mutexes use a signaling mechanism, where only one waiting thread is notified when the mutex becomes available, rather than all waiting threads.
📗 Locks and Priorities
Mutexes can also be used to prioritize certain threads over others. For example, a high-priority thread may be given exclusive access to a shared resource by acquiring a mutex, while lower-priority threads are blocked until the high-priority thread releases the mutex.
📗 Mutex vs Semaphores

Mutexes are best used for protecting a single shared resource, while semaphores are more suited for coordinating access to multiple shared resources. Semaphores can also signal multiple waiting threads, while mutexes can only signal one. Mutexes are simpler and more lightweight than semaphores, making them a better choice when only a single shared resource needs to be protected. On the other hand, semaphores are more powerful and flexible than mutexes, making them a better choice for more complex synchronization scenarios.
📗 Advantages of Using Mutexes
Mutexes provide several advantages in operating systems:
Synchronization: Mutexes provide a way to synchronize access to shared resources in a multi-threaded environment, preventing race conditions and other synchronization issues.
Simplicity: Mutexes are simple to implement and use, requiring only a few lines of code to acquire and release the mutex.
Efficiency: Mutexes are generally efficient, as they only block threads when necessary, allowing other threads to continue executing in the meantime.
Portability: Mutexes are widely supported across different operating systems and programming languages, making them a portable solution for synchronization.
📗 Disadvantages of Using Mutexes
While mutexes provide several advantages, they also have some disadvantages that should be considered:
Deadlocks: If not used properly, mutexes can lead to deadlocks, where multiple threads are blocked waiting for each other to release the mutex. This can cause the program to hang indefinitely, leading to a loss of functionality.
Overhead: Mutexes can add overhead to the program, as they require the operating system to manage and track the state of the mutex. This can lead to reduced performance and increased resource usage.
Priority inversion: Mutexes can also lead to priority inversion, where a low-priority thread holds a mutex that a high-priority thread needs to access. This can cause the high-priority thread to be blocked, leading to reduced system performance.
Code complexity: While mutexes are relatively simple to use, they can add complexity to the code, particularly when multiple mutexes are used to synchronize different resources.
📗 Producer Consumer problem
The Producer-Consumer problem is a classic synchronization problem in computer science that involves two or more processes or threads that share a common buffer. One process, called the producer, generates data and puts it into the buffer, while the other process, called the consumer, takes the data from the buffer and processes it. The problem is to ensure that the producer and the consumer do not access the buffer at the same time, which could result in data corruption or loss.
The producer-consumer problem can be described as follows:
There is a shared buffer that can hold a fixed number of items.
The producer generates items and puts them into the buffer.
The consumer takes items from the buffer and processes them.
The producer and consumer must synchronize their access to the buffer to avoid conflicts.
There are several solutions to the producer-consumer problem, including semaphores, mutexes, and monitors. Here, we will discuss two popular solutions: using semaphores and using mutexes.
✔ Using Semaphores
In this solution, two semaphores are used: one to keep track of the number of empty slots in the buffer (empty), and one to keep track of the number of items in the buffer (full).
The producer and consumer processes use these semaphores to synchronize their access to the buffer as follows:
When the producer wants to add an item to the buffer, it checks the value of the empty semaphore. If the value is greater than zero, it decrements the value of empty and adds the item to the buffer.
When the consumer wants to take an item from the buffer, it checks the value of the full semaphore. If the value is greater than zero, it decrements the value of full and takes the item from the buffer.
After the producer adds an item to the buffer, it increments the value of full to indicate that there is one more item in the buffer.
After the consumer takes an item from the buffer, it increments the value of empty to indicate that there is one more empty slot in the buffer.
✔ Using Mutexes
In this solution, a mutex is used to protect access to the buffer. Before accessing the buffer, the producer and consumer processes must acquire the mutex. After accessing the buffer, they release the mutex.
The producer and consumer processes synchronize their access to the buffer as follows:
Before adding an item to the buffer, the producer acquires the mutex. After adding the item to the buffer, it releases the mutex.
Before taking an item from the buffer, the consumer acquires the mutex. After taking the item from the buffer, it releases the mutex.
The advantage of using mutexes is that they provide a simple and efficient way to synchronize access to a shared resource. However, mutexes can lead to priority inversion and deadlocks if not used properly.
The producer-consumer problem is a common synchronization problem in operating systems and parallel programming, and there are many other solutions available. The choice of solution depends on the specific requirements of the application and the resources available on the system.
In summary, a mutex is a synchronization technique used in operating systems to prevent race conditions and other synchronization issues in a multi-threaded environment. It works by allowing only one thread to access a shared resource at a time, and is simple, efficient, and portable. While mutexes can lead to the thundering herd problem and may require careful handling of priorities, they provide an effective way to synchronize shared resources in a multi-threaded environment.
Thanks for reading, and happy coding!
Mutex and Producer-Consumer Problem: Synchronization Techniques in Operating Systems -> A Comprehensive Guide to Disk Management in Operating Systems