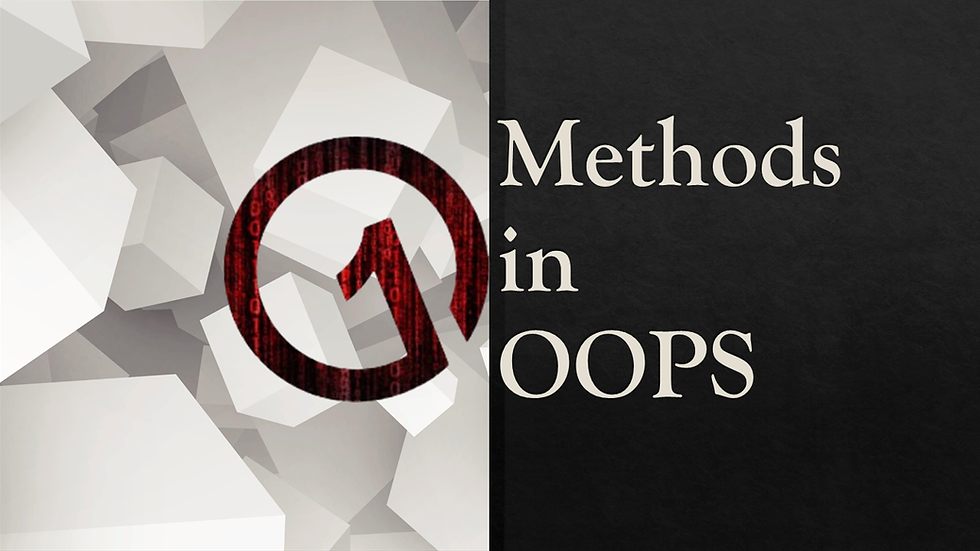
📗 Methods
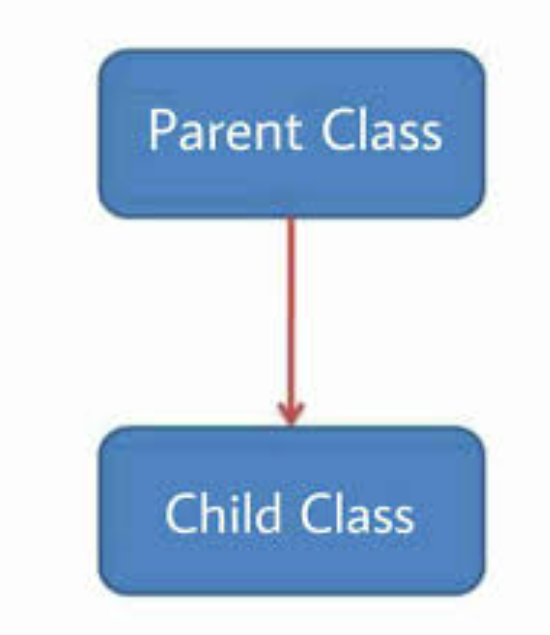
Methods are used to encapsulate a block of code and perform a specific task. They can be called from other parts of the program, which makes them a powerful way to reuse code. Methods in Java can take zero or more parameters, and they can also return a value.
Here's an example of a method that takes two parameters and returns the sum of those parameters:
public int add(int num1, int num2)
{
int sum = num1 + num2;
return sum;
}
Here's a line-by-line explanation of the code you provided:
public int add(int num1, int num2) {
This line declares a method called "add" that takes two integer parameters called "num1" and "num2". The "public" keyword means that the method is visible to other classes.
int sum = num1 + num2;
This line creates a new integer variable called "sum" and initializes it with the result of adding the values of "num1" and "num2".
return sum;
This line returns the value of the "sum" variable as the result of the method.
Overall, this code defines a method that takes two integers as input, adds them together, and returns the result as an integer.
For example, if you call this method with add(2, 3), it will return 5 because 2 + 3 = 5.
Calling a Method
you can call a method by using the name of the method followed by parentheses that contain any necessary arguments. Here's an example of how to call a method:
public class MyClass {
public void myMethod(int arg1, String arg2) {
System.out.println("arg1: " + arg1);
System.out.println("arg2: " + arg2);
}
public static void main(String[] args) {
MyClass myObject = new MyClass();
myObject.myMethod(42, "hello");
}
}
In this example, the MyClass class defines a method called myMethod that takes an integer argument called arg1 and a string argument called arg2. The method simply prints out the values of these arguments.
The main method of the class creates a new MyClass object called myObject using the new keyword. It then calls the myMethod method of the myObject instance by using the dot notation (myObject.myMethod) followed by the necessary arguments in parentheses.
When the program is run, it will output:
arg1: 42
arg2: hello
This is because the myMethod method was called with the arguments 42 and "hello", which were then printed out by the method.
Return Types of method
A method can have a return type, which indicates what type of value the method will return when it's called. The return type is specified in the method declaration, before the method name. Here's an example of a method with a return type:
public class MyClass {
public int add(int num1, int num2) {
int sum = num1 + num2;
return sum;
}
public static void main(String[] args) {
MyClass myObject = new MyClass();
int result = myObject.add(2, 3);
System.out.println("Result: " + result);
}
}
In this example, the add method takes two integer arguments and returns an integer value, which is the sum of the two arguments.
The main method creates a new MyClass object, calls the add method with the arguments 2 and 3, and stores the result in an integer variable called result. It then prints out the value of result.
When the program is run, it will output:
Result: 5
This is because the add method returned the value 5, which was stored in the result variable and then printed out by the main method.
Method Parameters
A method can take zero or more parameters, which are used to pass values into the method. Parameters are specified in the method declaration, within the parentheses after the method name. Here's an example of a method with parameters:
public class MyClass {
public void printMessage(String message) {
System.out.println(message);
}
public static void main(String[] args) {
MyClass myObject = new MyClass();
myObject.printMessage("Hello, world!");
}
}
In this example, the printMessage method takes a single parameter of type String, which represents the message to be printed. The method simply prints out the message using the System.out.println statement.
The main method creates a new MyClass object, and then calls the printMessage method with the argument "Hello, world!".
When the program is run, it will output:
Hello, world!
This is because the printMessage method was called with the argument "Hello, world!", which was then printed out by the method.
Standard Library Methods
There are many standard library methods that are built-in and can be used without having to create them from scratch. These methods are organized into different packages and can be accessed using import statements. Here are some examples of standard library methods:
Math.abs(x): Returns the absolute value of the argument x.
Math.max(x, y): Returns the maximum of the two arguments x and y.
Math.min(x, y): Returns the minimum of the two arguments x and y.
String.length(): Returns the length of the string.
String.charAt(index): Returns the character at the specified index in the string.
String.indexOf(str): Returns the index of the first occurrence of the specified string in this string.
Arrays.sort(array): Sorts the elements of the specified array in ascending order.
ArrayList.add(element): Adds the specified element to the end of the list.
File.exists(): Returns true if the file exists, false otherwise.
Scanner.nextLine(): Reads the next line of input from the user.
These are just a few examples of the many standard library methods available in Java. By using these methods, you can save time and effort by not having to create these functionalities from scratch.
what are the advantages of using methods ?
There are several advantages to using methods in Java:
Reusability: Methods allow you to write code once and reuse it multiple times in your program. This can save time and effort, and also makes your code more efficient and easier to maintain.
Modularity: Methods break down a program into smaller, more manageable pieces, making it easier to read, understand, and maintain. By breaking down a program into smaller components, you can more easily test and debug each individual piece.
Abstraction: Methods can hide complex implementation details and provide a simpler, higher-level interface for other parts of your program to use. This can help reduce complexity and make your code more modular and reusable.
Efficiency: Methods can help optimize code by allowing you to reuse code instead of writing it from scratch. This can help reduce duplication of code and make your program more efficient.
Readability: Methods make your code more readable by breaking it down into smaller, more easily understood pieces. This can make it easier for others to understand your code and for you to understand code written by others.
Overall, using methods in your Java programs can help improve your code's organization, efficiency, readability, and maintainability, while also making it easier to reuse and adapt your code for future projects.
📗 Method Overloading
Method overloading is a feature in Java that allows a class to have multiple methods with the same name but different parameters. This means that you can create multiple versions of a method with different input parameters, allowing you to perform similar tasks with different types of data.
The Java compiler determines which method to call based on the number and types of the parameters passed in. When a method is called, the compiler matches the parameters in the call with the parameters in each method declaration, and chooses the best match based on the number and types of the parameters.
Here's an example of method overloading:
public class MyClass {
public void printMessage(String message) {
System.out.println(message);
}
public void printMessage(int number) {
System.out.println("The number is: " + number);
}
public static void main(String[] args) {
MyClass myObject = new MyClass();
myObject.printMessage("Hello, world!"); // prints "Hello, world!"
myObject.printMessage(42); // prints "The number is: 42"
}
}
In this example, the MyClass class has two methods named printMessage, but with different parameters. One method takes a String parameter, and the other takes an int parameter. Both methods print a message to the console, but with different content based on the parameter.
When the program is run, it calls each of the printMessage methods with different parameters. The first call passes a String parameter, so the first printMessage method is called and prints "Hello, world!". The second call passes an int parameter, so the second printMessage method is called and prints "The number is: 42".
This is an example of method overloading, where two methods with the same name perform similar tasks, but with different types of input data.
Why method overloading?
Method overloading provides several benefits that make it a useful feature in Java:
Improved code readability: Method overloading allows you to create multiple methods with the same name that perform similar tasks with different input data. This can make your code more readable and easier to understand, as you don't need to come up with different names for each method that performs a similar task.
Better code organization: Method overloading can help you organize your code better by grouping related methods together with the same name. This can help improve code maintainability and make it easier to understand how different parts of your program work together.
Increased flexibility: Method overloading allows you to create methods that can handle different types of input data, which makes your program more flexible and adaptable to different use cases.
Reduced code duplication: Method overloading can help you reduce code duplication by creating methods that perform similar tasks with different input data. This can save time and effort, and make your code more efficient and easier to maintain.
Overall, method overloading provides a powerful and flexible way to write code that can handle different types of input data, while also improving code readability, organization, and maintainability.
⚡ How to perform method overloading in Java?
you can perform method overloading by creating multiple methods with the same name but different parameter lists. When a method is overloaded, the appropriate version of the method is selected based on the number, order, and types of the arguments passed to it. Here's an example of method overloading:
public class MyClass {
public int add(int num1, int num2) {
return num1 + num2;
}
public double add(double num1, double num2) {
return num1 + num2;
}
public String add(String str1, String str2) {
return str1 + str2;
}
public static void main(String[] args) {
MyClass myObject = new MyClass();
int intResult = myObject.add(2, 3);
System.out.println("intResult: " + intResult);
double doubleResult = myObject.add(2.5, 3.5);
System.out.println("doubleResult: " + doubleResult);
String stringResult = myObject.add("Hello, ", "world!");
System.out.println("stringResult: " + stringResult);
}
}
In this example, the MyClass class defines three versions of the add method, each with a different parameter list and return type:
The first version takes two integers and returns an integer sum.
The second version takes two doubles and returns a double sum.
The third version takes two strings and returns a string concatenation.
The main method creates a new MyClass object and calls each version of the add method with appropriate arguments. The appropriate version of the method is automatically selected based on the types of the arguments.
When the program is run, it will output:
intResult: 5
doubleResult: 6.0
stringResult: Hello, world!
This is because the appropriate version of the add method was called with the appropriate arguments, and the result was printed out.
Thanks for reading, and happy coding!
Methods in Object-Oriented Programming (OOP): types, advantage, usage -> Java Constructors: Creating Objects with Ease