
Getting Started...
Dart is a high-performance language that can be used for both client-side and server-side development. It is easy to learn and provides developers with a lot of powerful features that make it a great choice for building modern web applications.
To get started with Dart, you'll need to download and install the Dart SDK from the official Dart website. Once you have the SDK installed, you can use the Dart Editor or any other IDE that supports Dart to write and run Dart code.
To get started quickly, your best bet is to use the open-source tool DartPad, which lets you write and test Dart code via a web browser:
DartPad is set up like a typical IDE.
Some of the key components of DartPad are:
Code Editor: DartPad has a built-in code editor with syntax highlighting and automatic formatting, which helps developers write clean and readable code. The editor also provides features like code completion, error highlighting, and a command palette.
Console: DartPad has a console where developers can see the output of their code and debug any issues that arise. The console also provides access to the Dart Developer Tools, which can be used to profile and optimize the performance of Dart code.
Documentation: DartPad provides built-in documentation for the Dart language and standard library, making it easy for developers to learn and explore new features. The documentation is available in the form of an API reference, tutorials, and other resources.
Sharing: Developers can easily share their DartPad code snippets with others by generating a unique URL. This makes it a great tool for collaboration and learning.
Integration with other tools: DartPad can be used with other tools like Flutter and AngularDart, which makes it a great choice for developing web and mobile applications.
Why Dart?
DartPad is an online development environment for Dart that provides developers with a fast and easy way to write, run, and experiment with Dart code.
Some of the key features of DartPad include:
Real-time code execution: DartPad allows developers to see the results of their code changes in real-time, which makes it easy to experiment and test different ideas. Easy sharing: Developers can easily share their DartPad code snippets with others, making it a great tool for collaboration and learning. Built-in documentation: DartPad provides built-in documentation for the Dart language and standard library, making it easy for developers to learn and explore new features. Code highlighting and formatting: DartPad has a built-in code editor with syntax highlighting and automatic formatting, which helps developers write clean and readable code. Integration with other tools: DartPad can be used with other tools like Flutter and AngularDart, which makes it a great choice for developing web and mobile applications.
Overall, DartPad is a powerful and flexible tool that provides developers with a fast and easy way to experiment and learn the Dart language. Whether you're a beginner or an experienced developer, DartPad is a great way to explore the features and capabilities of Dart.
Core Concepts in DART
Dart programs begin with a call to main. Dart’s syntax for main looks similar to that of other languages like C, Swift or Kotlin.
Clear out all the code in the default DartPad and add main to the editor:
void main() {}
You’ll see there’s a return type before main. In this case, it’s void, meaning that main won’t return anything.
The parentheses after main indicate that this is a function definition. The curly brackets contain the body of the function.
Inside main, you add the Dart code for your program.
Next, you’ll learn more about the following core concepts:
Variables, Comments and Data Types
Basic Dart Types
Operators
Strings
Immutability
Nullability
Condition and Break
For Loops
Variables, Comments and Data Types -
Variables: Variables are used to store data in memory for later use in the program. In Dart, variables can be declared using the var keyword followed by the variable name and optionally a data type. For example, to declare a variable called name with the string value "John", you can write var name = "John";. You can also explicitly specify the data type, like String name = "John";.
Comments: Comments are used to document code and improve its readability. In Dart, single-line comments start with //, and multi-line comments are enclosed between /* and */. For example:
// This is a single-line comment in Dart/*
This is a multi-line comment
in Dart
*/
Data Types: In Dart, there are several built-in data types, including:
Numbers: Dart supports both integers and floating-point numbers. Integers can be specified using the int keyword, and floating-point numbers can be specified using the double keyword.
Strings: Strings are used to represent text in Dart. They can be created using either single quotes (') or double quotes (").
Booleans: Booleans are used to represent true/false values. In Dart, the bool keyword is used to specify a boolean variable.
Lists: Lists are used to store collections of objects. They can be created using square brackets [] and contain zero or more objects.
Maps: Maps are used to store key-value pairs. They can be created using curly braces {} and contain zero or more key-value pairs.
For example, to declare a variable of each data type, you can write:
int age = 30;
double price = 9.99;
String name = "John";
bool isTrue = true;
List<String> names = ["John", "Jane", "Bob"];
Map<String, int> ages = {"John": 30, "Jane": 25, "Bob": 40};
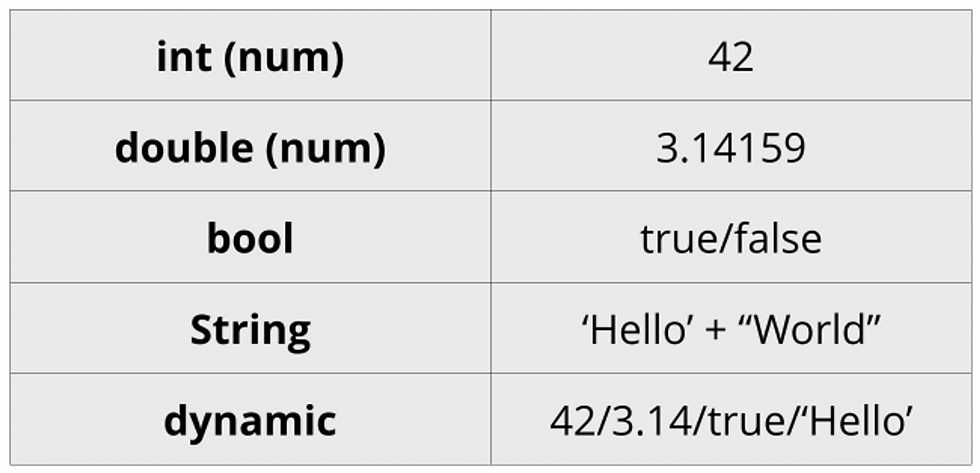
Overall, understanding variables, comments, and data types is crucial for writing effective and readable Dart code. By mastering these concepts, you can start building more complex programs in Dart.
The Dynamic Keyword
If you use the dynamic keyword instead of var, Here, you can set numberOfKittens to a String using quotes.
numberOfKittens = 'There are no kittens!';
print(numberOfKittens); // There are no kittens!
Run on dart pad to see the three different values for numberOfKittens printed in the console. In each case, the type of numberOfKittens remains dynamic, even though the variable itself holds values of different types.
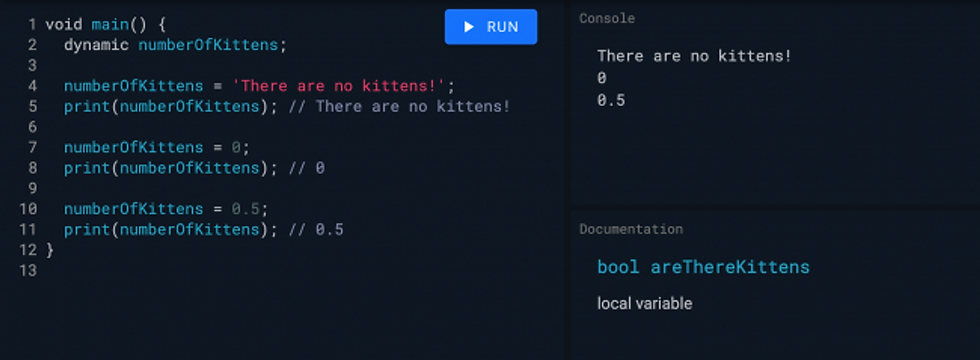
Booleans
The bool type holds values of either true or false.
Run the code again to see your Boolean values in the console. As given below -
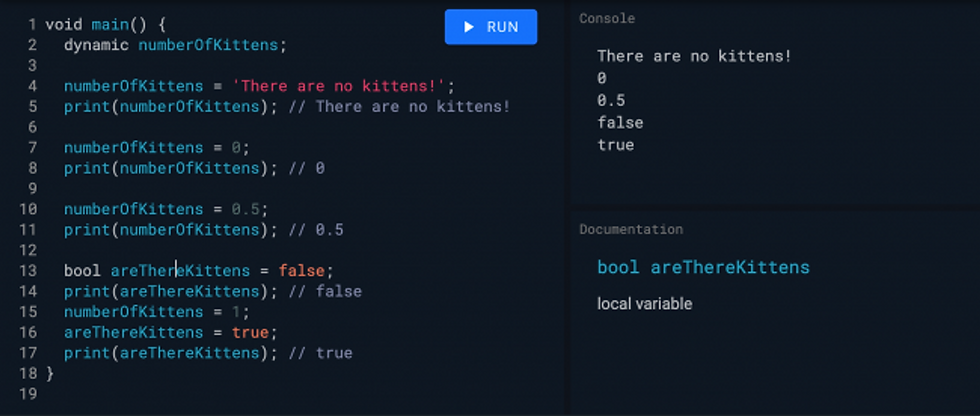
Operators
Dart supports several types of operators, each with its own set of rules and precedences. Here's an overview of each type:
Arithmetic Operators: Arithmetic operators are used to perform basic arithmetic operations on numbers. They include addition (+), subtraction (-), multiplication (*), division (/), integer division (~/), and remainder (%).
int a = 10;
int b = 5;
print(a + b); // 15
print(a - b); // 5
print(a * b); // 50
print(a / b); // 2.0
print(a ~/ b); // 2
print(a % b); // 0
Relational Operators: Relational operators are used to compare values and return a boolean result. They include equal to (==), not equal to (!=), greater than (>), less than (<), greater than or equal to (>=), and less than or equal to (<=).
int a = 10;
int b = 5;
print(a == b); // false
print(a != b); // true
print(a > b); // true
print(a < b); // false
print(a >= b); // true
print(a <= b); // false
Logical Operators: Logical operators are used to combine boolean values and return a boolean result. They include AND (&&), OR (||), and NOT (!).
bool a = true;
bool b = false;
print(a && b); // false
print(a || b); // true
print(!a); // false
Bitwise Operators: Bitwise operators are used to perform operations on binary values. They include AND (&), OR (|), XOR (^), NOT (~), left shift (<<), and right shift (>>).
int a = 10;
int b = 5;
print(a & b); // 0
print(a | b); // 15
print(a ^ b); // 15
print(~a); // -11
print(a << 1); // 20
print(a >> 1); // 5
Assignment Operators: Assignment operators are used to assign values to variables. They include simple assignment (=), addition assignment (+=), subtraction assignment (-=), multiplication assignment (*=), division assignment (/=), integer division assignment (~/=), remainder assignment (%=), and bitwise assignment (&=, |=, ^=, <<=, >>=).
int a = 10;
int b = 5;
a += b; // equivalent to a = a + b
a -= b; // equivalent to a = a - b
a *= b; // equivalent to a = a * b
a /= b; // equivalent to a = a / b
a ~/= b; // equivalent to a = a ~/ b
a %= b; // equivalent to a = a % b
a &= b; // equivalent to a = a & b
a |= b; // equivalent to a = a | b
a ^= b; // equivalent to a = a ^ b
a <<= b; // equivalent to a = a << b
a >>= b; // equivalent to a = a >> b
Strings
In Dart, a string is a sequence of characters enclosed in quotes, either single quotes or double quotes. Strings are a fundamental data type in Dart and are used extensively in programming.
Here are some of the key features and methods related to strings in Dart:
String interpolation: Dart allows you to insert values into a string using the ${} syntax. This makes it easy to build complex strings by combining variables and literals.
String productName = 'Widget';
int price = 10;
String description = 'Buy now and save!';
String message = 'The ${productName} is on sale for just \$${price}. ${description}';
print(message);
Output: The Widget is on sale for just $10. Buy now and save!
In this example, we use string interpolation to create a message that includes the product name, price, and description.
Concatenation: You can concatenate two or more strings using the + operator or the += shorthand.
String firstName = 'John';
String lastName = 'Doe';
String fullName = firstName + ' ' + lastName;
print(fullName);
Output: John Doe
Here, we concatenate two strings to create a full name.
Length: The length of a string can be obtained using the length property.
String message = 'Hello, world!';
int length = message.length;
print(length);
Output: 13
In this example, we use the length property to determine the number of characters in the message string.
Substrings: You can extract a substring from a string using the substring method. This method takes two arguments: the starting index and the ending index.
String message = 'Hello, world!';
String substring = message.substring(0, 5);
print(substring);
Output: Hello
In this example, we use the substring method to extract the first five characters of the message string.
Comparing strings: Strings can be compared using the == operator. This compares the contents of the strings, not the references.
String password = 'secret';
String confirmPassword = 'secret';
bool isMatch = (password == confirmPassword);
print(isMatch);
Output: true
Here, we use the == operator to compare two strings and determine if they are equal.
Converting to uppercase or lowercase: The toUpperCase and toLowerCase methods can be used to convert a string to uppercase or lowercase, respectively.
String message = 'Hello, world!';
String uppercaseMessage = message.toUpperCase();
print(uppercaseMessage);
String lowercaseMessage = message.toLowerCase();
print(lowercaseMessage);
HELLO, WORLD!
hello, world!
In this example, we use the toUpperCase and toLowerCase methods to convert a string to uppercase and lowercase, respectively.
Searching within a string: You can search for a substring within a string using the indexOf or lastIndexOf methods. These methods return the index of the first or last.
String message = 'Hello, world!';
int index = message.indexOf('world');
print(index);
Output: 7
Here, we use the indexOf method to search for the index of the substring "world" within the message string.
Escaping Strings
The escape sequences used in Dart are similar to those used in other C-like languages. For example, you use \\n for a new line. If there are special characters in the string, use \\ to escape them:
var quote = 'If you can\\'t explain it simply\\nyou don\\'t understand it well enough.';
print(quote);
This example uses single quotes, so it needs an escape sequence, \\', to embed the apostrophes for can’t and don’t into the string. You wouldn’t need to escape the apostrophe if you used double quotes instead. If you need to show escape sequences within the string, you can use raw strings, which are prefixed by r. Dart treat \\n as normal text because the string started with r.
Immutability
Dart uses the keywords const and final for values that don’t change.
Use const for values that are known at compile-time. Use final for values that don’t have to be known at compile-time but cannot be reassigned after being initialized.
Note: final acts like val in Kotlin or let in Swift.
You can use const and final in place of var and let type inference determine the type.
finalindicates that a variable is immutable, meaning you can’t reassign final values. You can explicitly state the type with either final or const:
final planet = 'Jupiter';
// planet = 'Mars';
// error: planet can only be set once
final String moon = 'Europa';
print('$planet has a moon, $moon');
// Jupiter has a moon, Europa
Nullability
In the past, if you didn’t initialize a variable, Dart gave it the value null, which means nothing was stored in the variable. As of Dart 2.12, though, Dart joins other languages, like Swift and Kotlin, to be non-nullable by default.
Additionally, Dart guarantees that a non-nullable type will never contain a null value. This is known as sound null safety.
Normally, if you want to declare a variable, you must initialize it:
String middleName = 'May';
print(middleName); // May
String? middleName = null;
print(middleName); // null
String? middleName;
print(middleName); // null
Not everyone has a middle name, though, so it makes sense to make middle Name nullable value. To tell Dart that you want to allow the value null, add ?after the type. The default for a nullable type is null, so you can simplify the expression as given above.
Null-Aware Operators
Dart has some null-aware operators you can use when you’re working with null values.
The double-question-mark operator, ??, is like the Elvis operator in Kotlin: It returns the left-hand operand if the object isn’t null. Otherwise, it returns the right-hand value:
var name = middleName ?? 'none';
print(name); // none
print(middleName?.length); // null
Since middleName is null, Dart assigns the right-hand value of 'none'.
The ?. operator protects you from accessing properties of null objects. It returns null if the object itself is null. Otherwise, it returns the value of the property on the right-hand side.
In the days before null safety, if you forgot the question mark and wrote middleName.length, your app would crash at runtime if middleName was null. That’s not a problem anymore, because Dart now tells you immediately when you need to handle null values.
Control flow
lets you dictate when to execute, skip over or repeat certain lines of code. You use conditionals and loops to handle control flow in Dart.
In this section, you’ll learn more about:
Conditionals
While Loops
Continue and Break
For Loops
Here’s what you need to know about control flow elements in Dart.
CONDITIONALS
If statements:
int age = 18;
if (age >= 18) {
print('You are old enough to vote.');
} else {
print('You are not old enough to vote.');
}
Output: You are old enough to vote.
In this example, we use an if statement to check if the age variable is greater than or equal to 18. If it is, the program prints a message indicating that the user is old enough to vote. Otherwise, it prints a message indicating that they are not.
Switch statements:
String color = 'red';
switch (color) {
case 'red':
print('The color is red.');
break;
case 'blue':
print('The color is blue.');
break;
default:
print('The color is not red or blue.');
break;
}
Output: The color is red.
Here, we use a switch statement to check the value of the color variable. If it is red, the program prints a message indicating that the color is red. If it is blue, the program prints a message indicating that the color is blue. If it is neither, the program prints a default message.
For loops:
for (int i = 0; i < 5; i++) {
print(i);
}
Output:
0
1
2
3
4
In this example, we use a for loop to print the numbers from 0 to 4.
While loops:
int i = 0;
while (i < 5) {
print(i);
i++;
}
Output:
0
1
2
3
4
This example shows how to use a while loop to print the numbers from 0 to 4.
Do-while loops:
int i = 0;
do {
print(i);
i++;
} while (i < 5);
Output:
0
1
2
3
4
Here, we use a do-while loop to print the numbers from 0 to 4.
Break and continue statements:
for (int i = 0; i < 5; i++) {
if (i == 3) {
break;
}
print(i);
}
Output:
0
1
2
In this example, we use the break statement to exit the loop when the i variable is equal to 3.
for (int i = 0; i < 5; i++) {
if (i == 3) {
continue;
}
print(i);
}
Output:
0
1
2
4
Here, we use the continue statement to skip the iteration when the i variable is equal to 3.
Collections
Collections are useful for grouping related data. Dart includes several different types of collections, but this tutorial will cover the two most common ones: List and Map.
Lists: A list is an ordered collection of objects, where each object can be accessed using its index. Lists can contain objects of different types.
List<int> numbers = [1, 2, 3, 4, 5];
print(numbers[2]); // Output: 3
numbers.add(6);
print(numbers); // Output: [1, 2, 3, 4, 5, 6]
In this example, we create a list of integers and access the element at index 2 using the square brackets. We also add a new element to the list using the add method.
Sets: A set is an unordered collection of unique objects. Sets can be used to remove duplicate objects from a list or to perform set operations such as union, intersection, and difference.
Set<String> fruits = {'apple', 'banana', 'orange', 'banana'};
print(fruits); // Output: {'apple', 'banana', 'orange'}
fruits.add('mango');
print(fruits); // Output: {'apple', 'banana', 'orange', 'mango'}
In this example, we create a set of strings and add four elements to it, including one duplicate element. The duplicate element is automatically removed when the set is created. We also add a new element to the set using the add method.
Maps: A map is a collection of key-value pairs, where each key is unique and maps to a corresponding value. Maps can be used to represent real-world entities such as a dictionary or a phonebook.
Map<String, String> phonebook = {
'Alice': '555-1234',
'Bob': '555-5678',
'Charlie': '555-9012',
};
print(phonebook['Bob']); // Output: 555-5678
phonebook['Dave'] = '555-3456';
print(phonebook);
// Output: {
Alice: 555-1234, Bob: 555-5678, Charlie: 555-9012, Dave: 555-3456
}
In this example, we create a map of string keys and string values representing a phonebook. We access the value for the key 'Bob' using square brackets. We also add a new key-value pair to the map using the square brackets and the = operator.
These are the three main types of collections in Dart, and they are widely used in Dart programming to group and manage data in a structured way.
Functions
Functions let you package multiple related lines of code into a single body. You then summon the function to avoid repeating those lines of code throughout your Dart app.
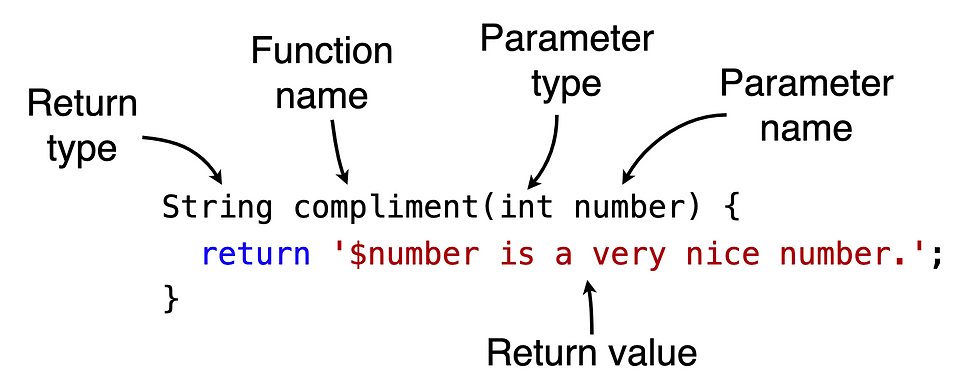
A function consists of the following elements:
Return type
Function name
Parameter list in parentheses
Function body enclosed in brackets
Defining Functions
The code you’re turning into a function goes inside the curly brackets. When you call the function, you pass in arguments that match the types of the parameters of the function.
Next, you’ll write a new function in DartPad that will check to see if a given string is banana:
bool isBanana(String fruit) {
return fruit == 'banana';
}
The function uses return to return a bool. The argument you pass to the function determines the bool.
This function will always return the same value type for any given input. If a function doesn’t need to return a value, you can set the return type to void. main does this, for example.
Working With Functions
You can call the function by passing in a string. You might then pass the result of that call to print:
void main() {
var fruit = 'apple';
print(isBanana(fruit)); // false
}
Nesting Functions
Typically, you define functions either outside other functions or inside Dart classes. However, you can also nest Dart functions. For example, you can nest isBanana inside main.
void main() {
bool isBanana(String fruit) {
return fruit == 'banana';
}
var fruit = 'apple';
print(isBanana(fruit)); // false
}
Optional Parameters
If a parameter to a function is optional, you can surround it with square brackets and make the type nullable:
String fullName(String first, String last, [String? title]) {
if (title == null) {
return '$first $last';
} else {
return '$title $first $last';
}
}
In this function, title is optional. It will default to null if you don’t specify it.
Now, you can call the function with or without the optional parameter:
print(fullName('Ram', 'Sharma'));
// Ram sharma
print(fullName('Shalini', 'Agarwal', 'Teacher'));
// Teacher Shalini Agarwal
Named Parameters and Default Values
When you have multiple parameters, it can be confusing to remember which is which. Dart solves this problem with named parameters, which you get by surrounding the parameter list with curly brackets: { }.
These parameters are optional by default, but you can give them default values or make them required by using the required keyword:
bool withinTolerance({required int value, int min = 0, int max = 10}) {
return min <= value && value <= max;
}
value is required, while min and max are optional with default values.
Run your code to see your new functions in action.
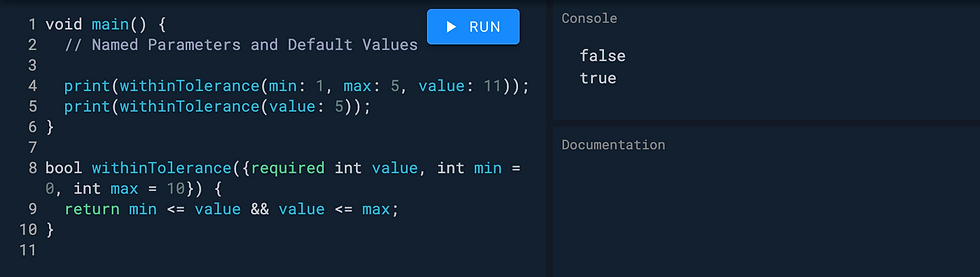
Thanks for reading, and happy coding!
Mastering Flutter Development Series Article - 4 -> Getting Started with Cross-Platform App Development : FLUTTER