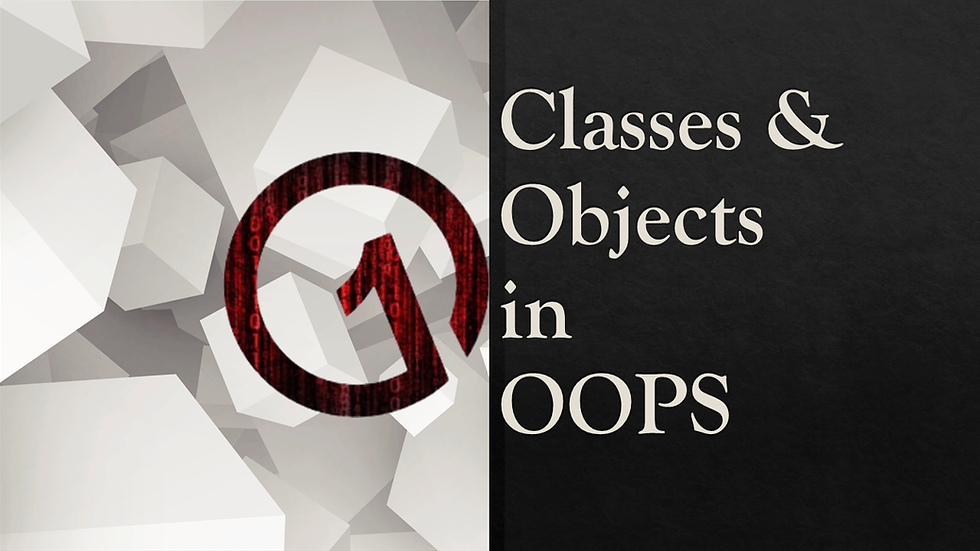
Object-oriented programming (OOP) is a programming paradigm that is centered around the concept of objects, which can be thought of as self-contained units of data and functionality. Classes and objects are the building blocks of OOP, and they are essential concepts that any programmer should understand.
Classes and objects can be a bit tricky to understand at first, but with practice and patience, they become second nature. In this explanation, I will go over the basics of classes and objects in OOP, and provide some tips and tricks for mastering them.
📗 Classes
In object-oriented programming, a class is a blueprint or template for creating objects. It defines a set of attributes and methods that the objects created from the class will have. Classes provide a way to organize code and define the behavior of objects.
The purpose of a class is to provide a structure for organizing code and data in a logical and reusable way. By defining a class, you can create objects based on that class and access its methods and attributes to perform specific tasks or operations. Classes can be thought of as a way to create custom data types that suit the needs of a particular program or application.
Create a class
We can create a class in Java using the class keyword. For example,
Here's an example of a simple class:
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
In this example, we've created a class called Person that has two attributes: name and age. The Person class also has a constructor that takes in a name and an age, and initializes the name and age attributes.
The Person class also has getter and setter methods for the name and age attributes. These methods provide a way to get and set the values of the attributes from outside the class.
📗 Objects
An object is an instance of a class. When you create an object, you are creating a specific instance of the class, with its own set of values for the attributes. You can think of an object as a concrete realization of the class blueprint.
Creating an Object
Define a class: First, you need to define a class that represents the type of object you want to create. A class is a blueprint or template that defines the properties and behaviors of an object.
public class Person {
// Properties
private String name;
private int age;
// Constructor
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// Behaviors (methods)
public void sayHello() {
System.out.println("Hello, my name is " + name + " and I am " + age + " years old.");
}
}
In this example, we've defined a Person class with a name and age property, a constructor to set those properties when a new object is created, and a sayHello() method to print a greeting.
Create an instance: Once you have defined a class, you can create an instance of that class by calling its constructor.
Person john = new Person("John Doe", 30);
This creates a new Person object and assigns it to the variable john. The constructor is called with the arguments "John Doe" and 30 to set the name and age properties of the new object.
Use the object: Now that you have an instance of the Person class, you can call its methods or access its properties as needed.
john.sayHello();
// Output: Hello, my name is John Doe and I am 30 years old.
This calls the sayHello() method on the john object, which prints a greeting to the console.
Overall, creating an object involves defining a class that represents the type of object you want to create, calling its constructor to create a new instance of that class, and then using the object by calling its methods or accessing its properties as needed.
Access Members of a Class
In Java, you can access members of a class using the dot notation (".") and the name of the member.
There are two types of members in a class: variables and methods. To access a variable, you need to use the dot notation followed by the name of the variable. For example, if you have a class called "Person" with a variable called "name", you can access it like this:
Person person = new Person();
String personName = person.name;
To access a method, you also need to use the dot notation followed by the name of the method, and include any necessary arguments in parentheses. For example, if you have a class called "Calculator" with a method called "add" that takes two integers as arguments, you can access it like this:
Calculator calculator = new Calculator();
int result = calculator.add(2, 3);
It's important to note that the accessibility of a member depends on its access level. If a member is declared as "private", it can only be accessed from within the class. If it's declared as "public", it can be accessed from anywhere. If it's declared as "protected", it can be accessed from within the class, subclasses, and classes in the same package. If no access level is specified, it's considered to have package-level access, meaning it can be accessed from within the same package.
Create objects inside the same class
In Java, you can create objects inside the same class by using the constructor of the class. Here's an example of how to create an object inside a class:
public class MyClass {
private int value;
public MyClass(int value) {
this.value = value;
}
public void createObject() {
MyClass object = new MyClass(10);
System.out.println("Created object with value " + object.value);
}
}
In this example, the createObject() method creates a new object of the MyClass class by calling the constructor with the value of 10. It then prints out the value of the newly created object.
Note that you can also create objects inside other methods or constructors of the same class. The process is the same - you just need to call the constructor with the appropriate arguments.
Tips and Tricks
Here are some tips and tricks for working with classes and objects in OOP:
Always use meaningful names for your classes and objects. Good naming conventions can make your code much easier to understand and maintain.
Keep your classes small and focused. Each class should have a single, well-defined responsibility.
Use encapsulation to protect the internal state of your objects. This means making data members private and providing public methods to access and modify them.
Use inheritance to create hierarchies of related classes. This can help you to reuse code and make your code more modular.
Use polymorphism to write code that can work with objects of different types. This can make your code more flexible and adaptable to changing requirements.
Always clean up after your objects. This means releasing any resources they have acquired (such as file handles or network connections) and freeing up memory when they are no longer needed.
Thanks for reading, and happy coding!
Mastering Object-Oriented Programming: A Comprehensive Guide to Classes and Objects in OOPs -> Feature/characteristics of OOPs.