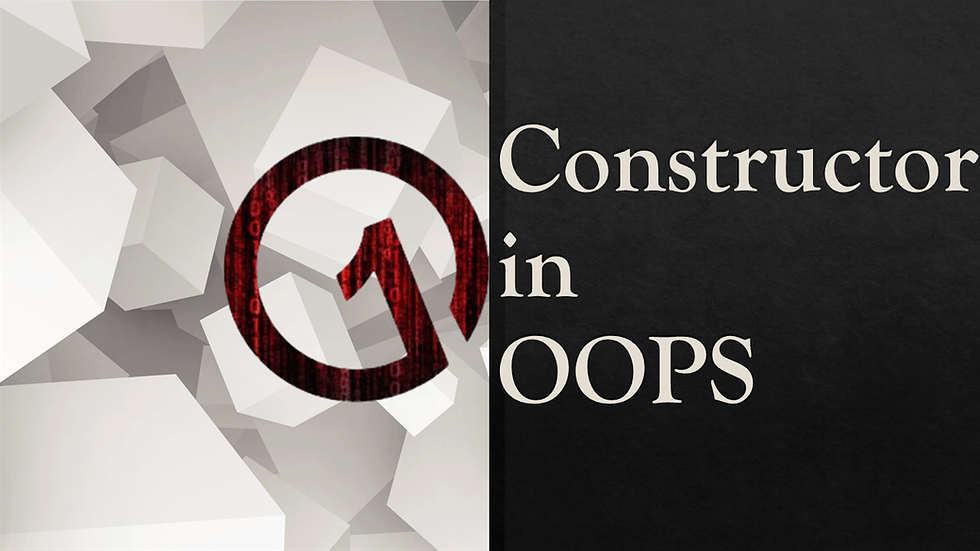
In Java, a constructor is a special method that is used to initialize objects when they are created. The constructor is called automatically when an object is created, and it can be used to set initial values for object properties or perform other setup tasks.
In general, a constructor has the same name as the class it belongs to, and it has no return type (not even void). Constructors can have parameters, which are used to pass data to the constructor when an object is created.
Here's an example of a simple constructor in Java:
public class MyClass {
private int myNumber;
public MyClass(int number) {
myNumber = number;
}
public int getNumber() {
return myNumber;
}
public static void main(String[] args) {
MyClass myObject = new MyClass(42);
System.out.println("The number is: " + myObject.getNumber());
// prints "The number is: 42"
}
}
In this example, the MyClass class has a single constructor that takes an int parameter. When an object of MyClass is created, the constructor is called automatically with the value 42, which is stored in the myNumber property of the object.
The MyClass class also has a getNumber method that returns the value of the myNumber property, and a main method that creates an instance of MyClass with the value 42, and prints the value to the console.
Constructors are an important part of object-oriented programming in Java, and they provide a convenient way to initialize objects when they are created.
Types of Constructors
In Java, there are four types of constructors:
Default constructor: This constructor has no parameters and provides a default set of values for object properties. If no constructor is defined in a class, Java automatically creates a default constructor.
Parameterized constructor: This constructor takes one or more parameters and uses them to set the initial values of object properties. The number and types of parameters depend on the specific needs of the class.
Copy constructor: This constructor creates a new object that is a copy of an existing object. It takes an object of the same class as a parameter and initializes the new object with the same values as the existing object.
Private constructor: This constructor is used to prevent the creation of objects of a class from outside the class. It is often used in singleton design patterns to ensure that only one instance of a class can exist.
Here's an example of each type of constructor in Java:
public class MyClass {
private int myNumber;
// Default constructor
public MyClass() {
myNumber = 0;
}
// Parameterized constructor
public MyClass(int number) {
myNumber = number;
}
// Copy constructor
public MyClass(MyClass other) {
myNumber = other.myNumber;
}
// Private constructor
private MyClass(int number1, int number2) {
myNumber = number1 + number2;
}
public int getNumber() {
return myNumber;
}
public static void main(String[] args) {
MyClass defaultObject = new MyClass(); // default constructor
// prints "Default number is: 0"
System.out.println("Default number is: " + defaultObject.getNumber());
// parameterized constructor
MyClass parameterizedObject = new MyClass(42);
System.out.println("Parameterized number is: " + parameterizedObject.getNumber()); // prints "Parameterized number is: 42"
MyClass copyObject = new MyClass(parameterizedObject); // copy constructor
System.out.println("Copy number is: " + copyObject.getNumber()); // prints "Copy number is: 42"
// Private constructor can only be called from within the class
// MyClass privateObject = new MyClass(10, 20);
// compile error
}
}
Constructors are an important part of object-oriented programming in Java, and understanding the different types of constructors can help you create classes that are more flexible and easier to use.
📕 Constructors Overloading
Constructors can also be overloaded in Java, just like regular methods. Constructor overloading allows you to create multiple constructors with different parameter lists, which can be used to create objects with different initial values.
Here's an example of constructor overloading in Java:
public class MyClass {
private int myNumber;
private String myString;
// Default constructor
public MyClass() {
myNumber = 0;
myString = "";
}
// Parameterized constructor with one parameter
public MyClass(int number) {
myNumber = number;
myString = "";
}
// Parameterized constructor with two parameters
public MyClass(int number, String string) {
myNumber = number;
myString = string;
}
public int getNumber() {
return myNumber;
}
public String getString() {
return myString;
}
public static void main(String[] args) {
MyClass defaultObject = new MyClass();
System.out.println("Default number is: " + defaultObject.getNumber());
// prints "Default number is: 0"
System.out.println("Default string is: " + defaultObject.getString());
// prints "Default string is: "
MyClass parameterizedObject1 = new MyClass(42);
System.out.println("Parameterized number is: " + parameterizedObject1.getNumber());
// prints "Parameterized number is: 42"
System.out.println("Parameterized string is: " + parameterizedObject1.getString());
// prints "Parameterized string is: "
MyClass parameterizedObject2 = new MyClass(42, "Hello, world!");
System.out.println("Parameterized number is: " + parameterizedObject2.getNumber());
// prints "Parameterized number is: 42"
System.out.println("Parameterized string is: " + parameterizedObject2.getString());
// prints "Parameterized string is: Hello, world!"
}
}
In this example, the MyClass class has three constructors: a default constructor that sets the initial values of the object properties to 0 and an empty string, a parameterized constructor with one parameter that sets the initial value of myNumber, and a parameterized constructor with two parameters that sets the initial values of myNumber and myString.
When an object is created, the appropriate constructor is called based on the number and types of arguments passed to the constructor. This allows you to create objects with different initial values based on your needs.
Constructor overloading is an important feature of Java, and it can help you create classes that are more flexible and easier to use.
Thanks for reading, and happy coding!
Java Constructors: Creating Objects with Ease -> Working with Strings in Java: Methods and Examples