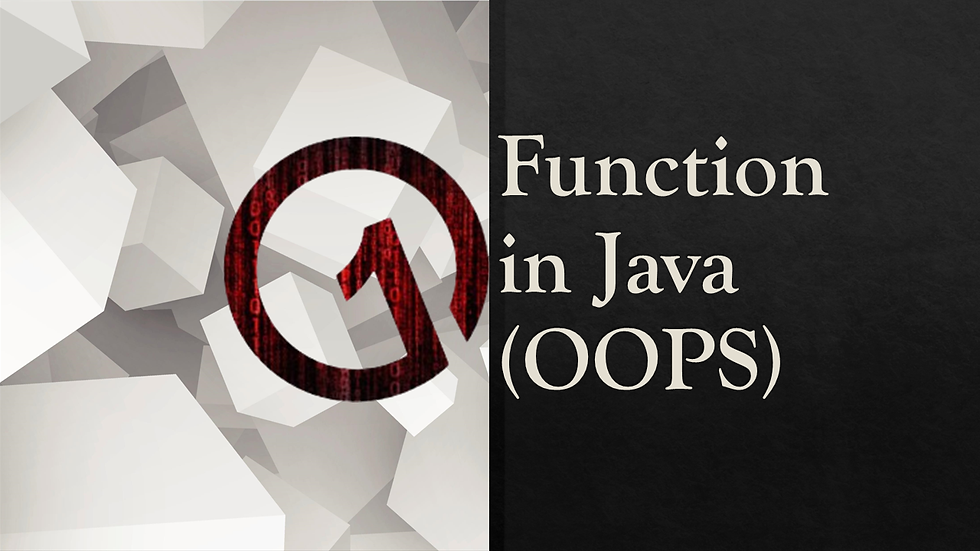
In Java, a function is called a method. A method is a block of code that performs a specific task and can be executed by calling it from another part of the program. Methods are used to modularize code, improve code readability, and facilitate code reuse.
Java methods have the following components:
Method Signature: The method signature is the combination of the method name and the parameter list. It specifies the name of the method and the type and order of the parameters that the method takes.
Method Body: The method body contains the code that is executed when the method is called.
Return Type: The return type specifies the type of the value that the method returns when it completes its execution.
📘 Important Functions
In object-oriented programming, the concept of "friend" refers to a mechanism that allows certain classes or functions to access the private and protected members of another class. In C++, there are friend functions and friend classes that provide this capability. However, friend functions and classes are not available in Java.
Here's a brief overview of how friend functions and friend classes work in C++:
âš¡ Friend Function
A friend function is a non-member function that is given access to the private and protected members of a class. This is achieved by declaring the function as a friend of the class in the class declaration. Here's an example:
class MyClass {
private:
int x;
friend void myFriendFunction(MyClass& obj) {
obj.x = 10;
}
};
In this example, the myFriendFunction() function is declared as a friend of the MyClass class. This allows the function to access the private member x of the class.
âš¡ Friend Class
A friend class is a class that is given access to the private and protected members of another class. This is achieved by declaring the friend class in the class declaration of the target class. Here's an example:
class MyClass {
private:
int x;
friend class MyFriendClass;
};
class MyFriendClass {
public:
void doSomething(MyClass& obj) {
obj.x = 10;
}
};
In this example, the MyFriendClass class is declared as a friend of the MyClass class. This allows the MyFriendClass class to access the private member x of the MyClass class.
In both cases, friend functions and friend classes provide a mechanism to break encapsulation and access the private and protected members of a class. While they can be useful in certain situations, they can also make the code more difficult to understand and maintain. In Java, access to the private and protected members of a class is controlled by access modifiers, such as private, protected, and public.
📘 Function Overriding vs Inline Function
Key Differences and Examples
Function overriding and inline functions are two important concepts in Java that can help you improve the performance and functionality of your code. Here's a brief overview of these two concepts, along with some examples to illustrate their differences.
âš¡ Function Overriding
Function overriding is a technique in object-oriented programming that allows a subclass to provide its own implementation of a method that is already defined in its superclass. This can be useful when you want to customize the behavior of a method for a particular subclass. Here's an example:
class Animal {
public void makeSound() {
System.out.println("The animal makes a sound");
}
}
class Cat extends Animal {
@Override
public void makeSound() {
System.out.println("The cat meows");
}
}
In this example, the Cat class overrides the makeSound() method that is defined in its superclass Animal. When the makeSound() method is called on a Cat object, it will execute the implementation provided by the Cat class, which prints "The cat meows" to the console.
âš¡ Inline Function
An inline function is a function that is expanded by the compiler at the point where it is called, rather than being executed as a separate function call. This can help improve the performance of the code by reducing the overhead of the function call. Here's an example:
public class MyClass {
public static int add(int x, int y) {
return x + y;
}
public static void main(String[] args) {
int result = add(3, 4); // This call will be inlined by the compiler
System.out.println(result);
}
}
In this example, the add() function is defined as a static method of the MyClass class. When the function is called with the arguments 3 and 4, the compiler will inline the function call and generate code that adds the two numbers directly, without executing a separate function call.
In summary, function overriding and inline functions are two different concepts that serve different purposes. Function overriding is used to customize the behavior of a method for a particular subclass, while inline functions are used to improve the performance of the code by reducing the overhead of function calls.
Thanks for reading, and happy coding!