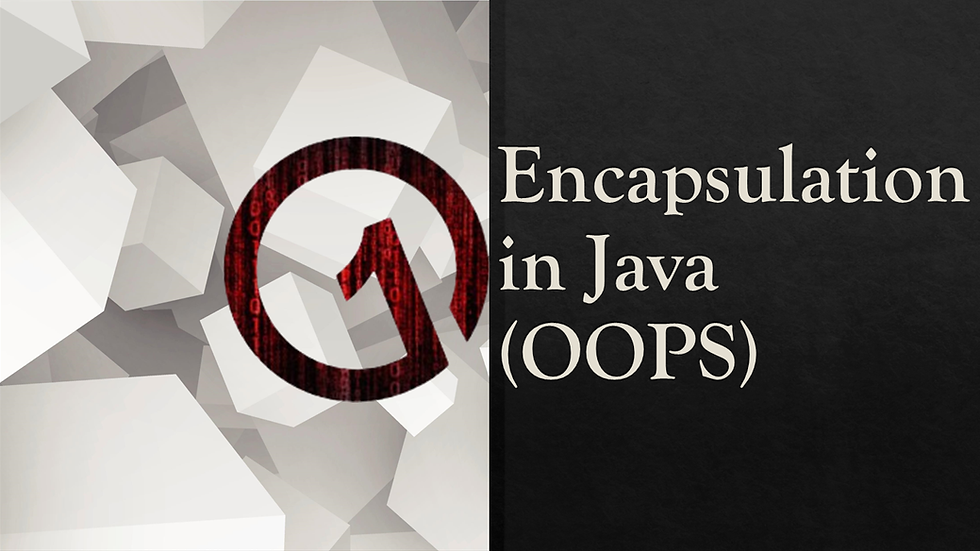
Encapsulation is one of the core principles of object-oriented programming (OOP) and is widely used in modern software development. It is a technique of hiding the implementation details of an object from the outside world and allowing access only to its behavior through a well-defined interface. Encapsulation is a key feature of OOP that promotes security, modularity, and maintainability of code. In this blog, we will define encapsulation, discuss its benefits, and provide examples of its implementation in Java.
📕 Definition of Encapsulation
Encapsulation is the process of wrapping data and methods within a single unit or object. The data is stored in private variables or fields, while the methods are defined as public or protected functions that operate on that data. The private fields are not directly accessible outside the object, and can only be accessed through the methods provided by the object. This way, the implementation details of the object are hidden from the outside world, and the object provides a well-defined interface for accessing its functionality.
Benefits of Encapsulation
Security: Encapsulation provides security by preventing unauthorized access to the object's internal data. By encapsulating the data within the object and restricting access to it, the object can ensure that the data is not modified in unintended ways, reducing the risk of bugs and errors.
Modularity: Encapsulation promotes modularity by breaking down a complex system into smaller, more manageable parts. Each object encapsulates its own data and methods, making it easier to understand, maintain, and modify the code. Encapsulation also promotes reusability by allowing objects to be used in different contexts without affecting their internal implementation.
Maintainability: Encapsulation makes code more maintainable by reducing the risk of unintended modifications to the object's internal data. Because the data is encapsulated, changes to the object's behavior can be made without affecting the rest of the system. This makes it easier to fix bugs and add new features to the codebase.
♦ Examples of Encapsulation in Java
Let's take an example of a class called "Employee" to illustrate the concept of encapsulation in Java. The class "Employee" contains two private fields, "name" and "salary", and two public methods, "getName()" and "getSalary()", to access these fields.
public class Employee {
private String name;
private double salary;
public Employee(String name, double salary) {
this.name = name;
this.salary = salary;
}
public String getName() {
return name;
}
public double getSalary() {
return salary;
}
}
In this example, the fields "name" and "salary" are private, meaning they can only be accessed by the methods within the "Employee" class. The public methods "getName()" and "getSalary()" provide a well-defined interface for accessing the data, allowing the user to retrieve the name and salary of an employee without accessing the private fields directly.
Encapsulation also allows for the implementation of setters and getters, which are methods that allow for the modification of private fields. The setter method is used to modify the value of a private field, while the getter method is used to retrieve the value of a private field. This allows the object to control how its data is modified and accessed, promoting the principles of encapsulation.
public class Employee {
private String name;
private double salary;
public Employee(String name, double salary) {
this.name = name;
this.salary = salary;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getSalary() {
return salary;
}
public void setSalary(double salary) {
this.salary = salary
}
}
Encapsulation is implemented in Java using access modifiers, which control the visibility and accessibility of the class members (fields and methods) from outside the class. Java provides three types of access modifiers: private, protected, and public.
♦ Private access modifier:
Fields and methods declared as private are accessible only within the same class.
This provides a way to hide the implementation details of a class and prevent direct access to its internal state from outside the class.
Access to private members is possible through public methods of the class, called getters and setters, which provide controlled access to the class's fields.
♦ Protected access modifier:
Fields and methods declared as protected are accessible within the same class, its subclasses, and classes in the same package.
This provides a way to implement inheritance and to control access to the class's members from its subclasses and other classes in the same package.
♦ Public access modifier:
Fields and methods declared as public are accessible from any class, anywhere in the program.
This provides a way to expose the class's interface to the outside world and to allow access to its members from other classes.
♦ Default access modifier:
In Java, if no access modifier is specified for a class, method, or variable, it is assigned the default access modifier.
The default access modifier is also known as package-private, and it means that the class, method, or variable can only be accessed within the same package.
♦ Advantages of Encapsulation:
Data Hiding: Encapsulation allows data to be hidden and accessed only through public methods, which provide controlled access to the class's fields. This prevents direct access to the class's internal state and helps to maintain the integrity and consistency of the data.
Modularity: Encapsulation provides a way to modularize a program by grouping related data and methods into a single unit, called a class. This makes it easier to understand and maintain the code and promotes code reusability.
Inheritance: Encapsulation is essential for implementing inheritance in object-oriented programming. It allows the subclass to access the protected and public members of the superclass and provides a way to reuse the code and extend the functionality of the superclass.
Polymorphism: Encapsulation is also essential for implementing polymorphism in object-oriented programming. Polymorphism allows objects of different classes to be treated as objects of a common superclass, which is possible only if the superclass's interface is exposed through public methods.
📕 Advance Example of Encapsulation
Now, let's look at an advanced example of encapsulation in Java. Here, we'll create a class called BankAccount that represents a bank account, with private fields for accountNumber, balance, and customer, and public methods to deposit and withdraw funds, check the balance, and get customer information.
public class BankAccount {
private String accountNumber;
private double balance;
private Customer customer;
public BankAccount(String accountNumber, double balance, Customer customer) {
this.accountNumber = accountNumber;
this.balance = balance;
this.customer = customer;
}
public void deposit(double amount) {
balance += amount;
}
public void withdraw(double amount) {
if (balance >= amount) {
balance -= amount;
} else {
System.out.println("Insufficient balance");
}
}
public double getBalance() {
return balance;
}
public Customer getCustomer() {
return customer;
}
}
In this example, the accountNumber, balance, and customer fields are declared as private, so they can only be accessed and modified from within the BankAccount class. The constructor initializes these fields with values passed in as parameters.
The public deposit and withdraw methods provide controlled access to the balance field from outside the class, allowing funds to be added or removed from the account. The getBalance method returns the current balance of the account.
The getCustomer method returns the Customer object associated with the account, allowing information about the account holder to be retrieved.
In summary, encapsulation is a fundamental concept in Java that allows data and methods to be bundled together into a single unit, called a class, and controlled access to its members from outside the class using access modifiers. Encapsulation promotes data hiding, modularity, inheritance, and polymorphism, and is an essential part of object-oriented programming.
Thanks for reading, and happy coding!
Encapsulation in Object-Oriented Programming (OOP): Definition, Benefits, and Examples. ->
Mastering Java Nested and Inner Classes: Understanding the Differences and Benefits